I got the idea for a game a few days ago. I started prototyping it using the DOM and absolutely positioned elements before I realized I was better off using proper tools for the job. As such, I decided to dust off a book I bought a couple of years ago and write my own 2D game engine, which I’m calling “Patina”.
A while back, I taught full stack web development at a successful startup. In order to keep students engaged, we taught DOM manipulation by having them implement various video games, including all of the following:
While the first two are a good fit for using the DOM for graphics, the latter two would arguably have been better off implemented using the Canvas API. Since game development was technically outside of the scope of the course – that is, it was a means to an end – we stuck with DOM manipulation.
For my game idea (the details of which, I’ll write about another time), I figured I could take the same short cut to implement it. Indeed, I could have, but I quickly remembered all the pain points of absolute positioning of elements and trying to animate those using CSS. Prototype or not, I didn’t enjoy writing the game because of these pain points. As such, I decided to take a step back and use a proper graphics framework.
All of the rabbit holes
For a while, I considered writing the game in a language that compiled to WebAssembly, such as in C using RayLib or in Rust using Bevy. Either seemed interesting enough, but I really wanted to be able to write games in a functional way.
I thought back to some of my early game development experiments years ago. A lot of the frameworks I was looking into followed the same general pattern that React eventually adopted: Model-View-Update.
The basic idea is that your
model
represents the state of the game at any given time, an update
function
takes as input a model
representing the previous frame of your game, as well
as an action
and returns a new model
using the old one. Finally, a view
function renders the state of your game.
Sound familiar? You’ve seen this before if you ever played with redux.
You can see a working example of this idea in Elm’s Mario Demo;
Suffice it to say, I never found anything that quite ticked all of the boxes for me (Elm as a language is inadequate for other reasons). Then I took a look at my bookshelf and realized that I could eventually, hopefully, make something myself.
To shave a yak
The book in question is “Build Your Own 2D Game Engine and Create Great Web Games”.
It steps through the process of making your own 2D game engine using the aforementioned canvas API and WebGL. My (possibly naive) plan, was thus:
- Learn to write a low-level 2D game engine that runs games on the web
- Make a few simple games in the process, not unlike I had my students do with the DOM API
- Implement a more declarative, functional layer on top of that.
- (Finally) implement my game!
After a couple of chapters, I now know a little bit about vertex and fragment shaders, as well as how to set up a context to draw on. Several lines of TypeScript later, and I’m able to draw a colored rectangle on a colored canvas:
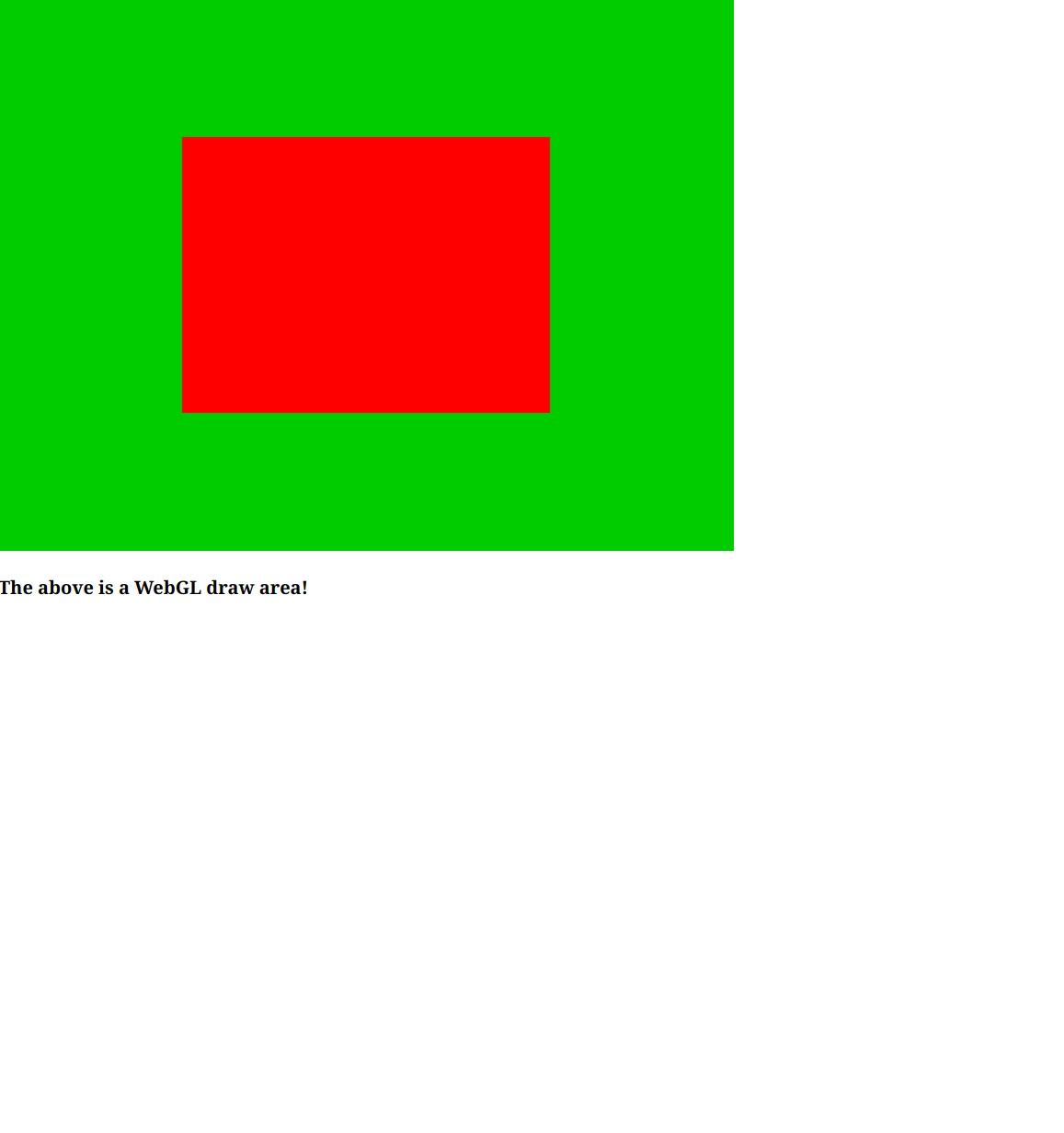
If you’d like to take a look at the code I’ve written up until this point, feel free to check out the git repository.